Your First Request
Running your first API request is a little like writing a 'Hello World!' program when learning something like C or Java. It is a simple example that demonstrates some of the basic principles involved. With that in mind, we will run our first request. As mentioned previously, the API needs to be running as described in the previous section. If it isn't still running, you can run it again and you should see the message, Listening on Port 3000 as shown in figure 3.
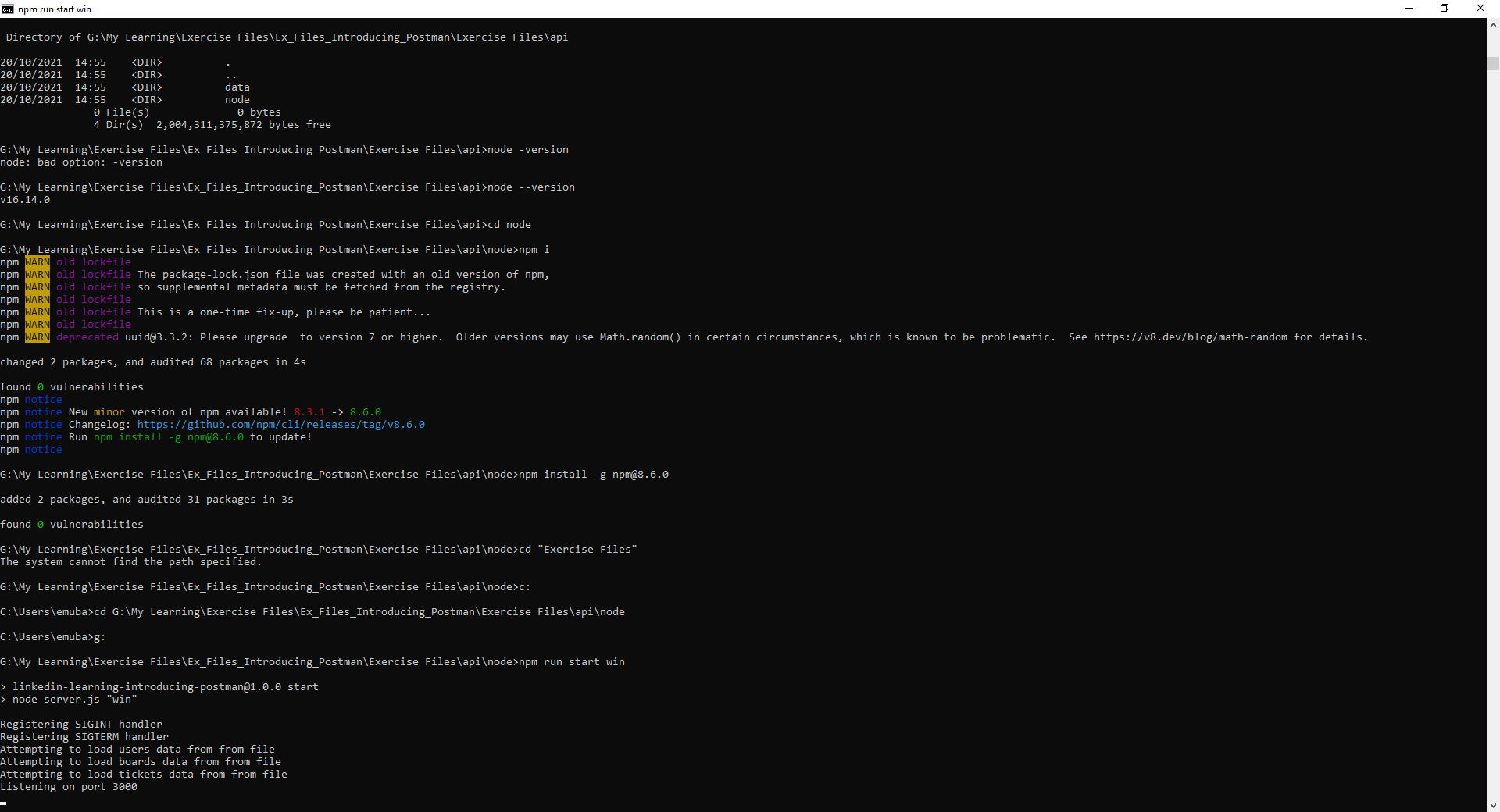
Since the API is running locally and listening on port 3000, we will start by entering the URL
http://localhost:3000
Everything in the sample API is routed under api so we will add that to the URL to give us
http://localhost:3000/api
Next, we need to specify the HTTP method for our request. If we click the drop-down menu next to the method (which is get by default), this displays a list of methods that are available to you. Some of these are standard methods and some are specific to different APIs, but the most common methods are listed at the top and these incldude GET, POST and PUT. In this case, we will select the GET method.
We can also define our own methods in Postman but that is beyond the scope of this course.
There are several ways for us to send the request. The simplest way is to simply click the Send button. We can also use the keyboard shortcut Control + Enter or select the drop down menu on the Send button and select Send and Download. This can be a useful option if you need to inspect the results later. In this case, I have selected Send and Download and the response is saved as an HTML file which can be viewed by clicking here.
If you get an error when you try to send the request, there are a few things you should check.
• Is the API running, in this case our sample API
• Have we used the correct URL
• Have we used the correct HTTP verb, GET
Assuming all goes well, we should see the output as shown in figure 4.
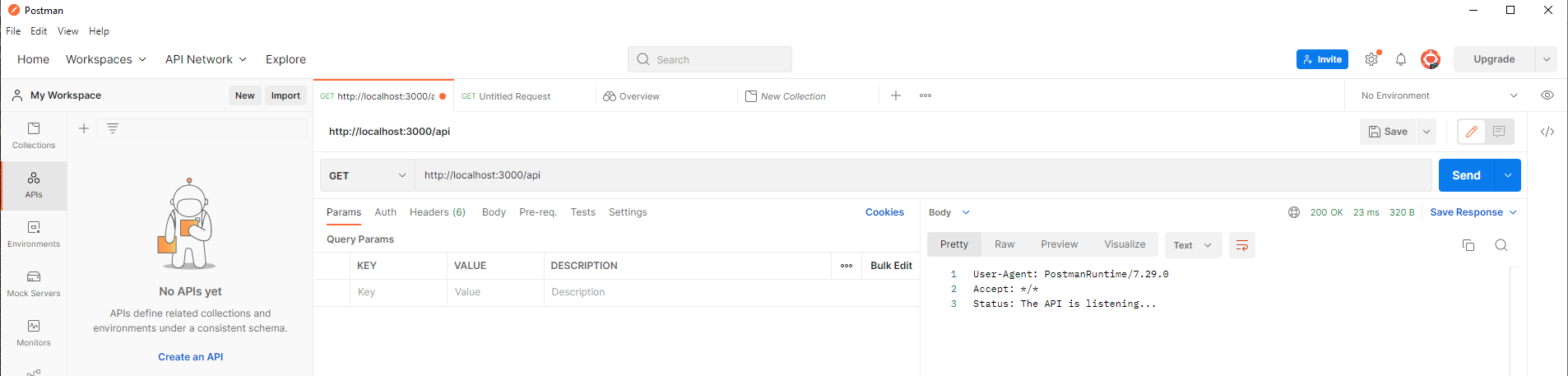
Notice that with the output, we have different options for its presentation. The default is Pretty. If the output has a known structure such as json or xml, pretty will try to apply formatting to make it look neater. The Raw option will just give you the raw data without any formatting. The Preview option will attempt to render the output and is useful if you want to know how that will look in a browser.
Note that in some instances, these options will not be available. For instance, if the output is an image file, Postman will just try to render it rather than let the user decide how the output should be displayed.
Going back to the pretty option as seen in figure 4, there is also a drop down menu which currently shows Text and this is the content type. This is selected automatically by Postman and you will normally find that it is correct, but you can change it here if you need to. In some (possibly most) cases, selecting another option will not really make much sense so you won't see much difference, if any at all. In this case, the output is simple text so selecting an option such as json will try to format the results as json which partly changes the texy colour which I guess is Postman identifying key value pairs. We can ignore the option here, but with different output, we may see much greater differences.
In addition to viewing the reponse body, which we are doing here, we can also view the headers and cookies. Since I have a more recent version of Postman that the one David is using in the course videos, I see a couple of other options as well and cookies is shown as a link which opens up a box with info relating to cookies. In this example, there are no cookies so there is nothing for us to see there.
We will see some data listed under headers. In figure 4, you can see that there are 6 headers and unlike David's version, my version of Postman hides auto-generated headers by default but if you click the button that shows the number of headers (6 in figure 4), the headers are then visible. In the course video, there are 7 and comparing these two sets of headers, one is the same on both, there is also one that looks similar, but the rest are different. This may be because of the different versions of Postman we are using but I think that it is more likely because of the different platforms. I belive David is using a Mac rather than a Windows desktop which is what I am using. In this case, the headers are pretty basic so I'm not going to worry too much about them at the moment.
Postman also displays some additional info when we run a request which confirms the status, time taken and size of the response. Again, we can see this in figure 4 which shows a status of 200 indicating that the response was succesfully returned. We can also see that it took 23ms and had a size of 320 bytes.
Manage Query String Paramters
Query string paramters are commonly used when we want to pass some data such as search terms over to an API. A good example of this can be found when you try to run a search on Google. For example, I tried searching for "query string paramters example" (in this case, the search terms are not important, they are just used to demonstrate the process) and when I click search, this gives me the following URL.
https://www.google.com/search?q=query+string+parameters+example&newwindow=1&sxsrf=......
I have truncated this because we are really only interested in what is shown after the Google address. We mentioned before when composing our first request that everything was being routed under API and in this example, it is being routed through search. For Google, the search terms are added to the URL using the q paramter (it also appends some other parameters for tracking purposes).
To see how this works, we are going to use Postman to get a list of boards from the API. You can start a new request by pressing Control and T (on a Windows machine, this would be Command and T on a Mac) and insert the same URL as before but with boards added to the end.
We will start with the same base URL, so
http://localhost:3000/api
We will append baords to the end of that to give
http://localhost:3000/api/boards
If we send that, we will see the output shown in figure 5.
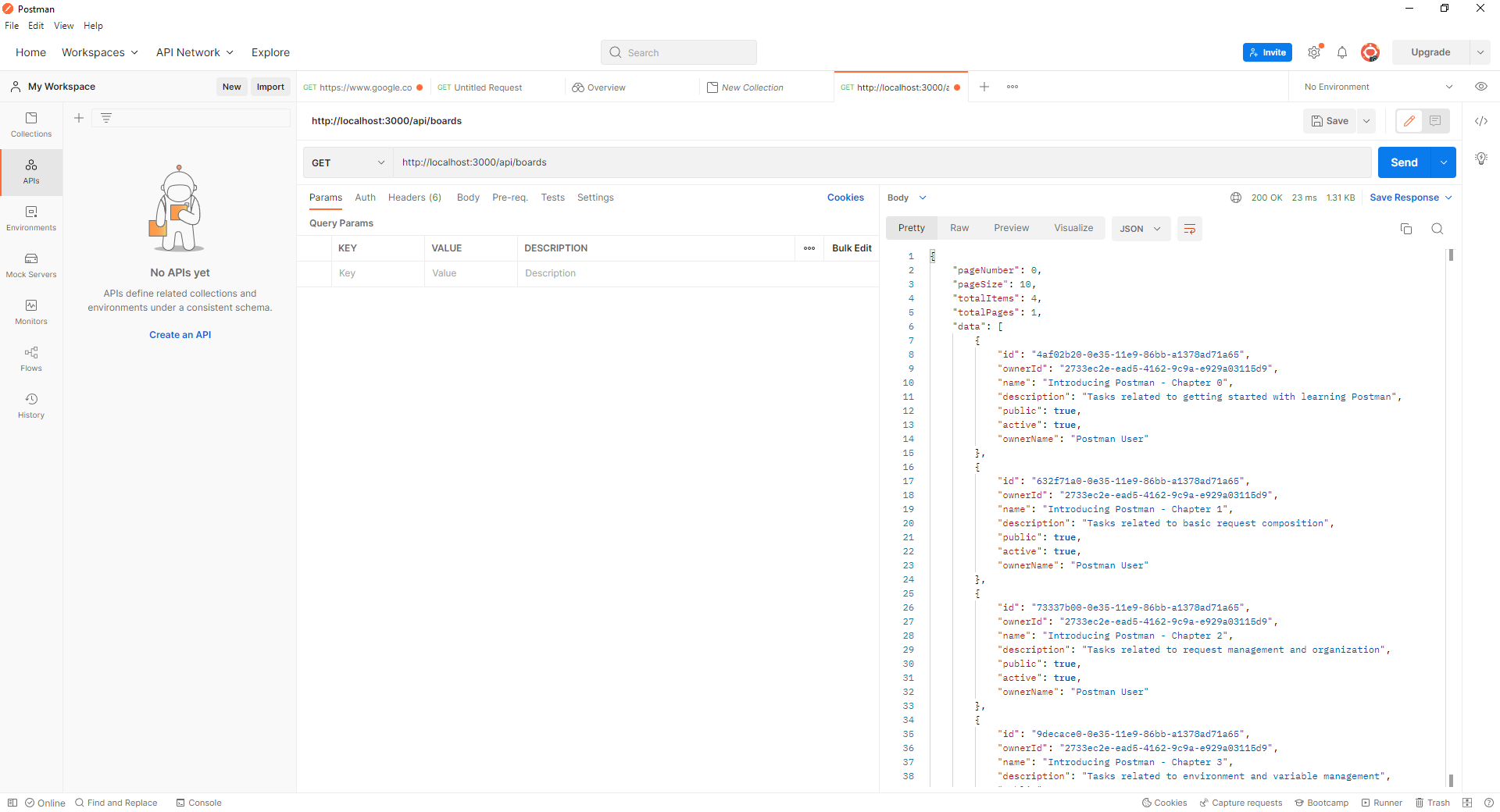
SIDE NOTE: When I initially sent th request, I didn't get any data returned and this turned out to be down to the way I had started the api with the command, npm run start win. This should be
npm run start-win
When I sent the request again, I got the output shown in figure 5.
The output provides us with some data so we have a page number starting at 0, page size which is 10, total items which is 4 and total pages which is 1. These are all default values and we will see how these can be changed in a moment. This is followed by the data relating to the boards that we had requested.
Let's say that we want to change the page size so that we see only one of those boards on each page. We can do that with a string paramter, which for page size is ps. This can be appended to the URL with a question mark so this becomes
http://localhost:3000/api/boards?ps=1
This gives us the output shown in figure 6.
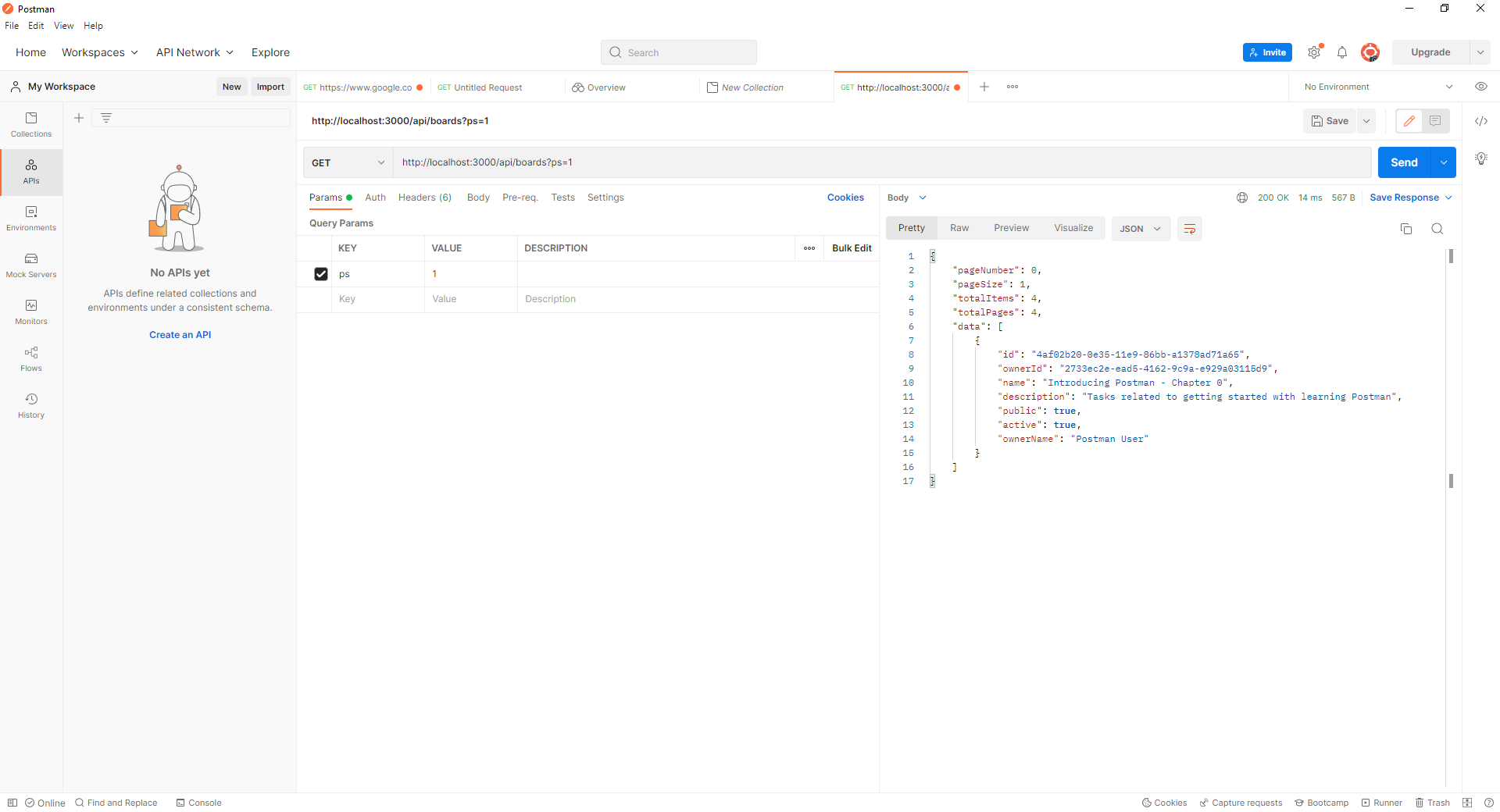
As you can see, we now have a page size of 1 and as there are four boards, this means that we have four pages.
If you are appending string parameters to the URL because if you are using a URL reserved character, that has to be encoded so let's say that you wanted to add a parameter called This & That but Postman does help you with that. To add a second string parameter, we will separate this from the first paramter with that same ampersand character (which is why it is reserved) so let's start by amending the URL like this
http://localhost:3000/api/boards?ps=1&This & That
If we send the request as it is, Postman will interpret both of those ampersand characters as separators and will therefore see this as three string paramters. Since it doesn't recognise what it sees as the second and third of those, it ignores them. If we select both the parameters and right-click, we can select the option, EncodeURIComponent which will do the encoding for us and change the URL to
http://localhost:3000/api/boards?ps=1&This%20%26%20That
Note that the ampersand has been encoded as %26 and we also have two characters which have been encoded as %20. These are the spaces surrounding the ampersand and we could have just left those out. As a matter of interest, you can also do these translations online at url-encode-decode.com which also provides some additional useful information about URL encoding and includes a list of characaters that are allowed in the URL along with a list of the reserved characters which you will need to encode. For reference, these are
! * ' ( ) ; : @ & = + $ , / ? % # [ ]
If we do have a URL with encoded characters, we can also decode them by selecting the URL, or at least the part that includes the encoded characters, right-clicking and selecting DecodeURIComponent
As before, if we send this, the additional paramter (note that now the URL is properly encoded, Postman recognises the fact that we only have two parameters) is ignored but I have shown the output of this in figure 7.
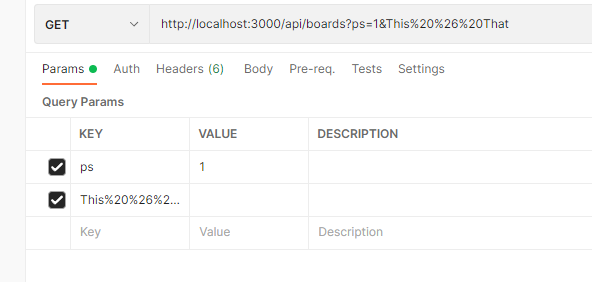
Notice that we are displaying the query parameters. To make this a little clearer, figure 4 shows a zoomed in view of the query parameters.
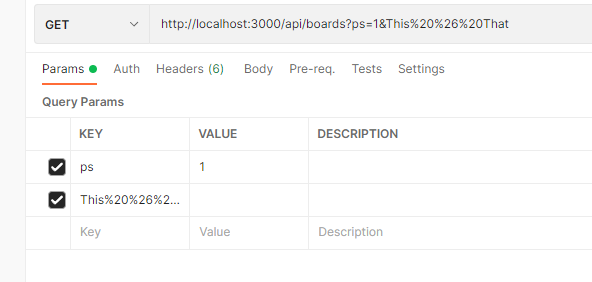
Note that the This & That parameter also appears here and this shows us a second way to add query parameters to the request. We can add the parameter itself as the key and its value. For example, let's say we want to remove the This & That parameter and change the page size to 2. The This & That parameter can be removed by clicking the X at the end of its description box. We can also untick the parameter so that it is not deleted but is removed from the URL. To change the page size to 2, we can simply change the number under VALUE. Note that as we make these changes, the URL is automatically updated to reflect these changes so that is another and perhaps easier way to add query parameters to the URL.
This is known as the key-value editor and as we have seebn, it works in both directions so we can update the paramters displayed there by editing the URL or we can update the URL by editing the parameters in the key-value editor.
The key-value editor also provides some other useful functions such as disabling a parameter as we saw with This & That and you can, of course, enable paramters by selecting them. We can also reorder the parameters using the handle that appears if you hover over one of the parameters (this is shown in figure 8).
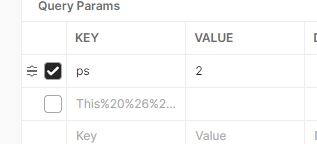
You can use this by simply clicking on it and moving the parameter to the desired position.
You can add other parameters using either method. For exampe, in the key-value editor, we can insert pn as the key and give it a value of, for instance 3. The revised URL now looks like this.
http://localhost:3000/api/boards?ps=1&pn=3
If we resend the request, we still see the same results as before with 4 pages, each displaying board. This time, the output starts at page 3.
You can also edit the query parameters by clicking on Bulk Edit in the key-value editor. This is a toggle so when the Bulk Edit window is open, clicking it again will take you back to the key value editor. If we disable the secondquery paramter and then click Bulk Edit, the result hould be something like that shown in figure 9.
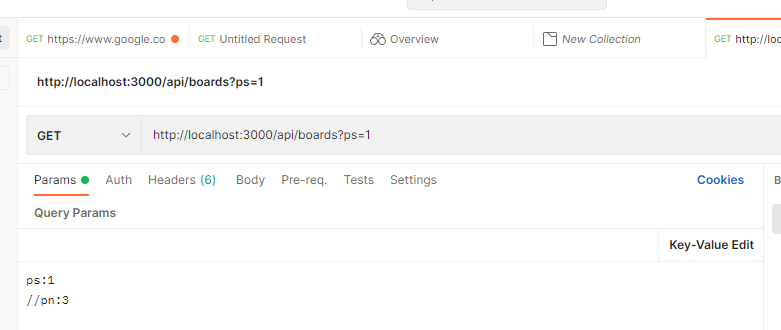
Notice that the pn parameter is shown there, but it is commented out. Bulk Edit uses a JavaScript like syntax so
Parameters are separated with a new line
The key an value are separated by a colon
Parameters can be disabled by adding // to the start of the line
Manage Cookies
None of the requests we have looked at so far have involved cookies, so if you click on the Cookies link, you will see a message stating that there are no cookies. If you create a new request using GET and the URL, linkedin.com, when you send the request, the web page is returned (the sign in page) and because this site uses cookies, we can now see them in Postman and this is shown in figure 10.
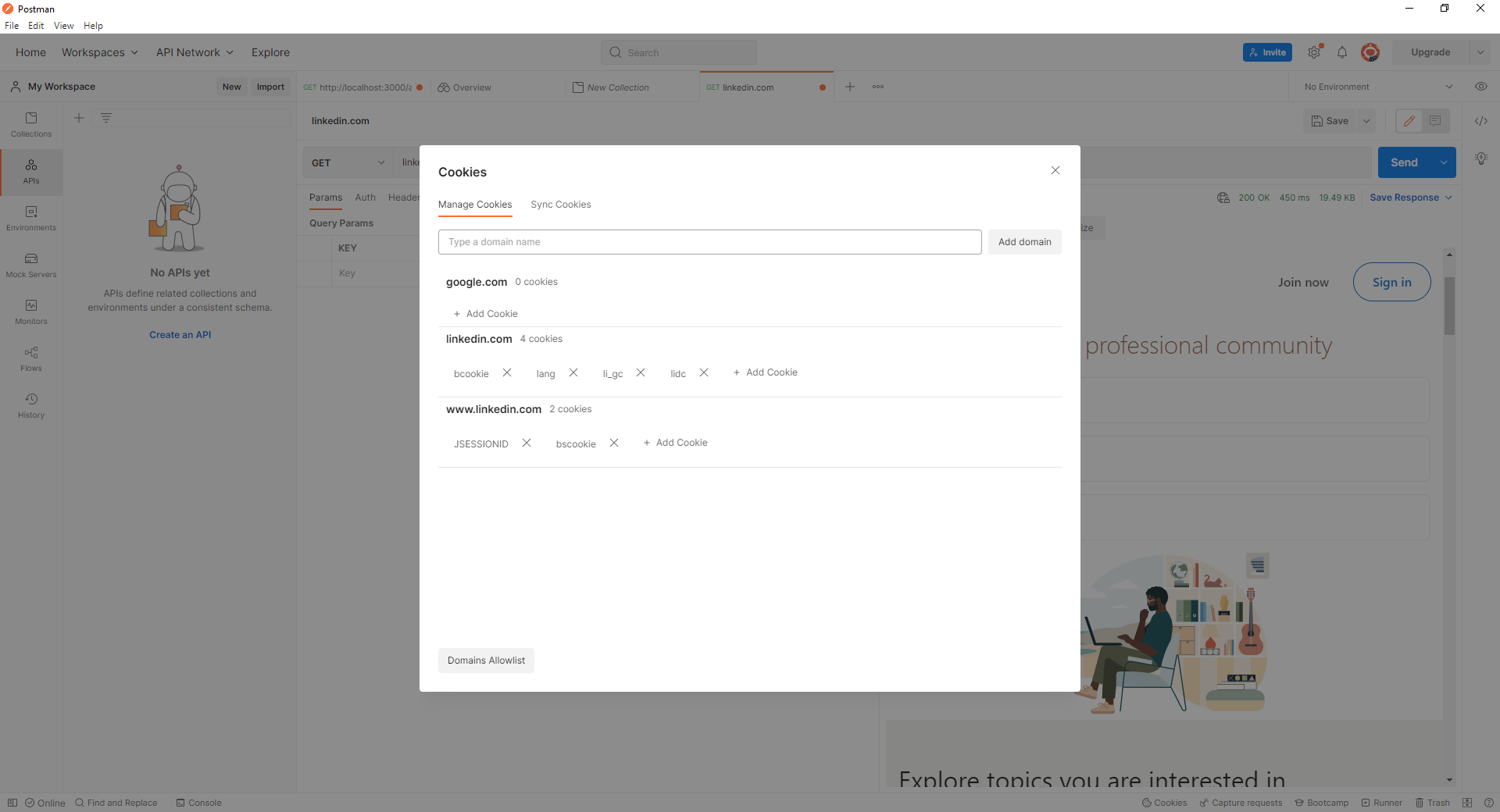
The image in figure 11 shows the Manage Cookies dialog with the mouse hovering over the title bar for the linkedin.com cookies and you can see there is an X there which you can use to close all of the cookies in the group or you can close cookies individually.
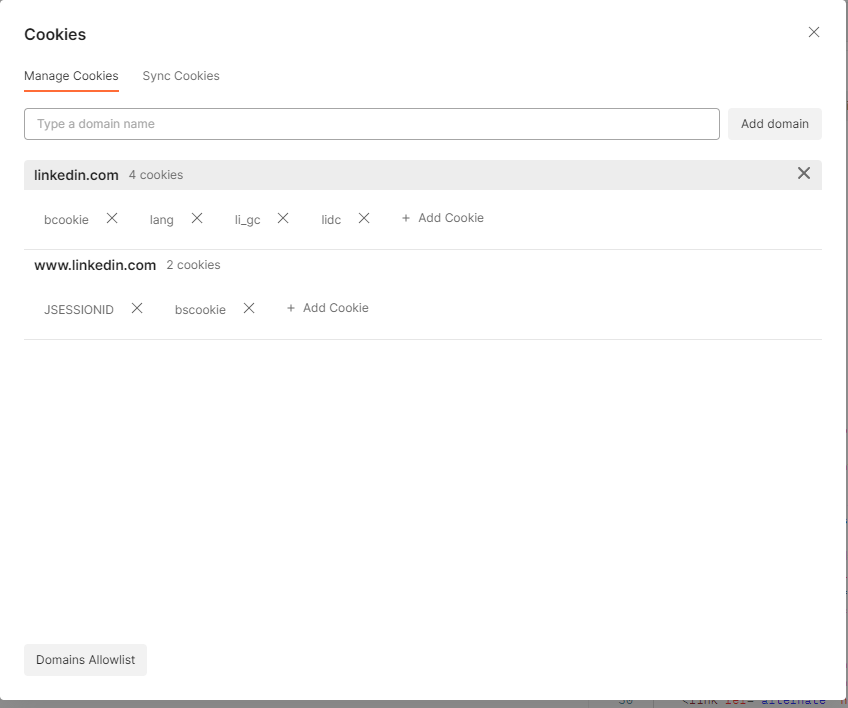
We can click on the name of one of the cookies to give us access to edit it. We can see an example of this in figure 12 where we are looking at the lang cookie for LinkedIn. Notice that this a lang property with a value of en-us. If we change the value to es-mx and resend the request, then click the preview button, we see the login page again but it is displayed in Spanish.
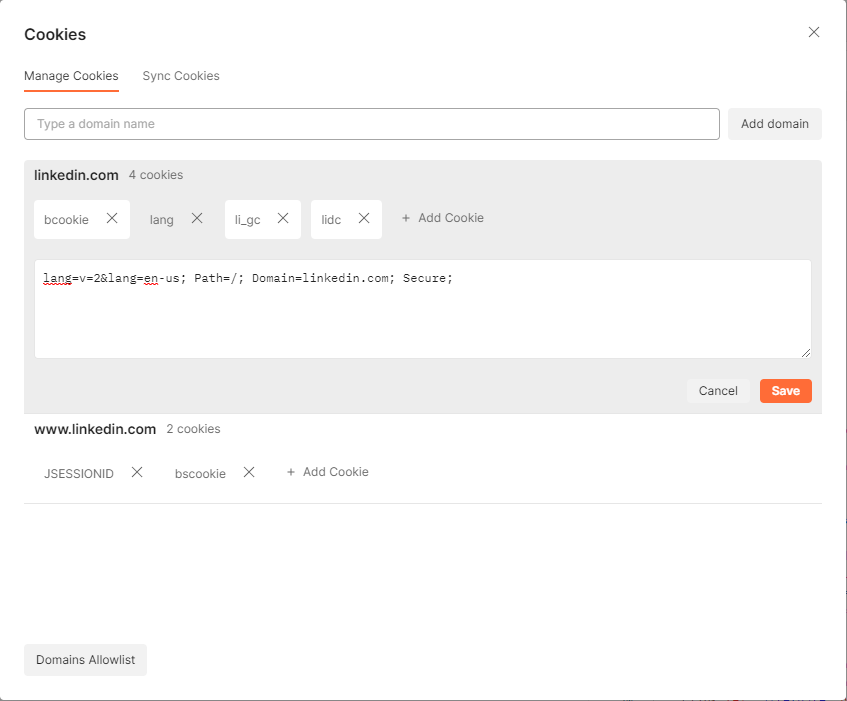
We can control other aspects of the cookie here such as the name, the path, the domain and so on.
Manage Request Headers
Request headers are used to pass additional information to the API or website and this information can be used to determine how to respond to the request. Examples of this include
Accept header
The accept headers are used to determine the format in which a response should be sent.
Authorization header
The authorization header is used to manage access to content.
User-Agent header
The user-agent header is used to pass over information about where the request is coming from.
To illustrate this, we will go back to our first GET request
http://localhost:3000/api
and we want to look at the body. This is shown in figure 13 and bear in mind that the headers here have not been edited at all, these are the default values.
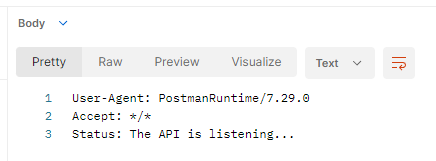
Note that the user-agent header identifies the sending afent as the PostmanRuntime version 7.29.0 and the accept header effectively let's the API know that we will accept the response in any format.
If we want to edit the request headers, there is an editor similar to the one we saw earlier when editing parametrs and we also have a bulk edit option, so the process of editing these is the same. One advantage when editing the request headers is that there is a fairly well-defined set of these. This means, for example, that if you start typing the header in the key column, you will see that there are likely to be auto-suggestions for the header you are looking for. You can see this by typong Us in the key and that is enough to show User-Agent in the suggestions list. If we select that, we can then add a custom value for the User-Agent header and we will do that here by entering linkedin/postman as the value.
We already had a user-agent header set, but adding the key again results in the default header being disabled (however, we can't edit the existing header directly so we do need to add it again in order to provide a new value). If we send the request, we see the custom user-agent appearing in the results we get when sending the request. This is shown in figure 14.
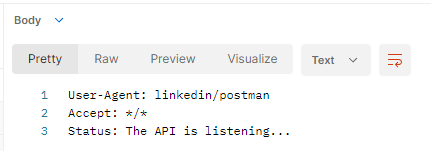
We can similarly add a new accpet header and we will give it a value of application/json - again, this will appear in the list of auto suggestion. If we then send the request again, we will see that the response is now in the json format as shown in figure 15.
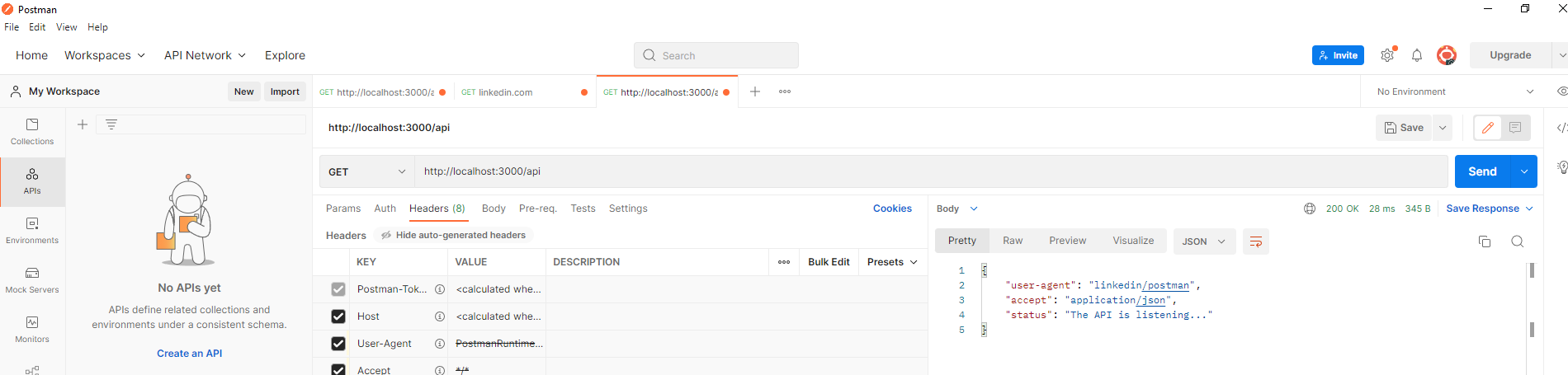
You can compare this to the data we saw being returned in figure 4.
Manage Headers Presets
As the name suggests, a header preset is a group of headers we can save and call up in order to use the same headers and values for another request. In the previous section, we set a couple of headers, the user-agent (with a value of LinkedIn/Postman) and accept (with a value of application/json). We will now save these in a preset.
We can see the header editor in image 14 above. Next to the link to Bulk Edit, there is a Presets dropdown menu. If you don't have any presets defined, there will only be one menu item and that is Manage Presets. If you do have some presets defined, these will also be listed in that menu. Similarly, if you select Manage Presets, this will open the dialog box shown in figure 15.
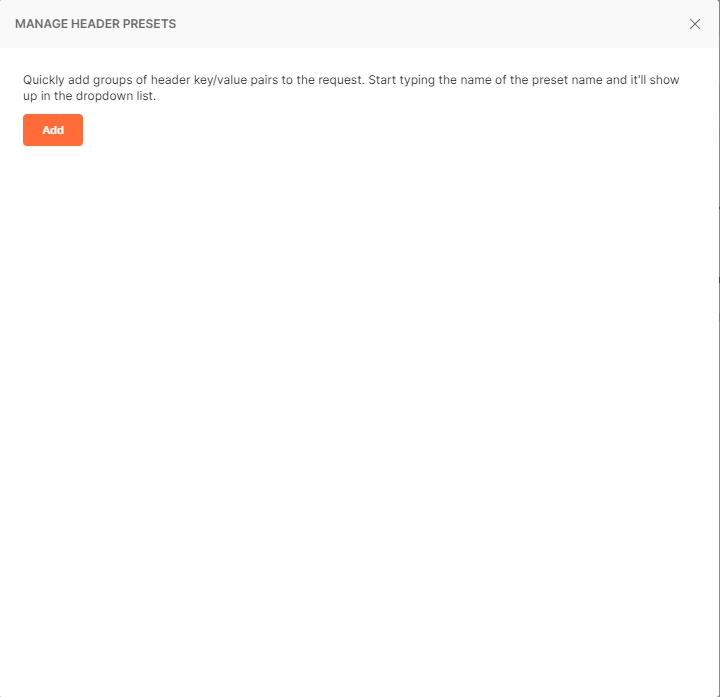
We can click the Add button to set up and we will call the preset LinkedIn + JSON and we will add the same two presets in the keys and values as shown in figure 16.
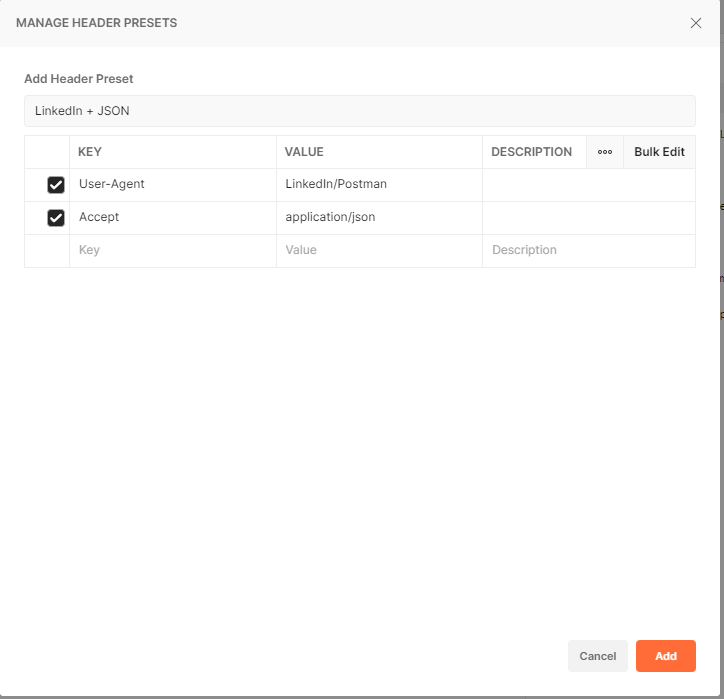
We can then click on the Add button to complete the process of creating our first preset. Now, we will see this in the Presets drop-down menu as well as in the Manage Presets dialog.
In order to see how it works, you will want to make sure that you don't already have these headers set so make sure that if they are still present, you delete them. We can then select the Preset from the drop down menu and we can then see that the preset headers are present. We can also send the request again and see that they are both shown in the headers editor. We can also see that these have the desired effect in that the user-agent is shown in the results and the format is JSON, as shown in figure 17.
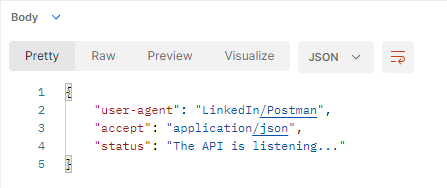
With preset headers, it is important to remember that when you select a preset, the headers are copied to the request rather than merged which means that there is a possibility that some if the headers may be duplicated. It also means that if you change the presets, the changes are not automatically reflected in a request where you had previously copied a preset. There are advantages and disadvantages to this.
Firstly, this means that we are not dependent on the preset but it also means that making changes can be more difficult because if you want to use the modified preset, you have to copy it into the request again and possibly also delete existing geaders to avoud any possible conflict.
Build a Request Body
The request body is another way in which we can send data to an API and is very useful for building such things as a search query. To illustrate this, we will send a request to the sample API so we will be using the same URL as before, routing the request against boards and adding search so the basic URL is
http://localhost:3000/api/boards/search
Remember that the requests we have sent so far were asking the API to return something, we haven't really passed any data to it other than headers to control the response. Another way to look at it is that we have sent a request that returns a specific response and we also sent some additional parameters which have no effect on what is returned, only on how it is formatted. For that, a GET request is fine.
When we add a request body, we are asking the API to return something specific. We are asking it to perform a search and return the results, so we have to use an appropriate HTTP method that allows us to include a bodt. In this case, the API specifies that we use a the POST method.
If you click on the Body tab, you might notice that the text area, where we create the body is not editable because we have to select the format in which we want to send the data to the server. The format you choose will depend on the API and what its requirements are. As an example, a simple file upload might expect data as form fields in which case we would select form-data which gives us the type of editor we are now familar with although it is slightly different here. For example, if you click on the key field, a drop-down menu appears where we can select the data type - text or file. This is shown in figure 18.
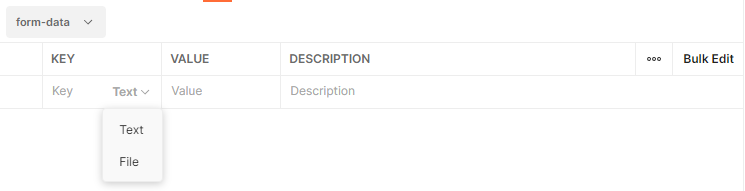
If we select file as the data type, a button appears in the value field allowing us to select the file and this is shown in figure 19. Clicking this will open a standarad Windows File Open dialog which we can use to navigate to and select the required file.
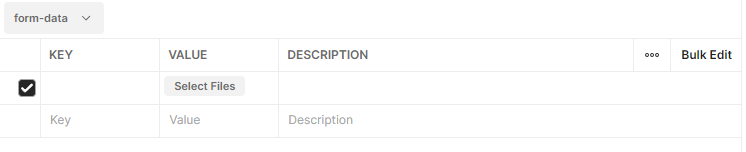
There is also an option to send our data in the form, x-www-form-urlencoded which is similar to form-data. For our search, we will be sending JSON so we want to select the raw option. This makes the text editable and that's where we will type the JSON to configure our search. You might notice that when you select Raw, you see the word Text appearing next to it with a drop-down menu, as shown in figure 20. This is the default option for raw data so before we start, we should change this to JSON.
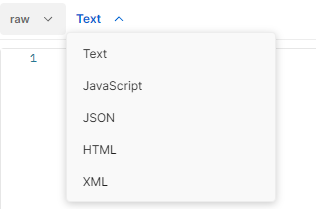
Note that the JSON type is shown as application/json (this is true of the version of Postman in the course video, in my version it only shows as JSON) which we saw earlier with the request headers, specifically in reference to the accept header. Setting the accept header to a value of application/json would have exactly the same effect as selecting JSON here so you can do that either way, but selecting it from the drop-down menu is probably the easiest.
Note that if we send the request as it is, without typing anything in the body, we get everything returned so the JSON we are going to add will have the effect of filtering these search results. In the text area, we will input the JSON with a couple of attrbiutes, the first of which is filters. That is, the key wwill be "filters" and the value will be an array with two attrbutes, a "field" with a value of "description" and a "value" with a value of "management". This is specifying that we want to search for something in the descritopn field and the value we want to search for is management.
The second attribute we want to add is "sort" which simply has a value of "name" since we want our results to be sorted by name. So this gives us the body shown in figure 21.
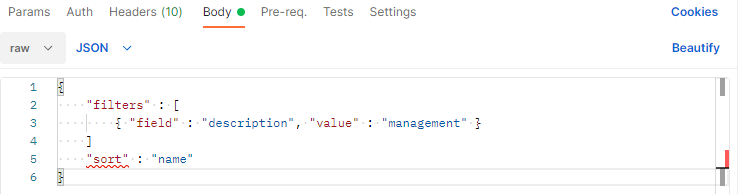
Notice that there is a beautify link and this is one of the advantages of selecting the data type here. If you switch back to Text as the data type, the Beautify link will disappear.
Switch back to JSON and the link reappears. If we click it, this will reformat our JSON into a more standardised form as shown in figure 22.
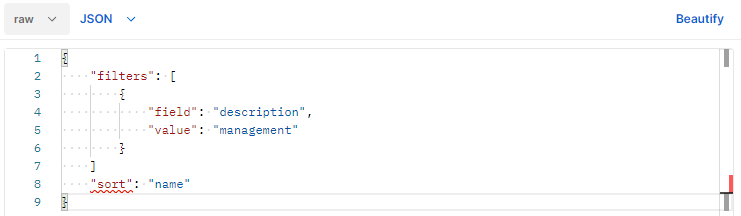
When you send the request, you might get a response in HTML which usually indicates an error in the JSON somewhere. For example, you might have noticed a squiggly red line under the word, sort, which you might think means there is a spelling error, but I will come back to that in a second - spoiler, it's not a spelling error!
We will go ahead and run this and the results are show in figure 23.
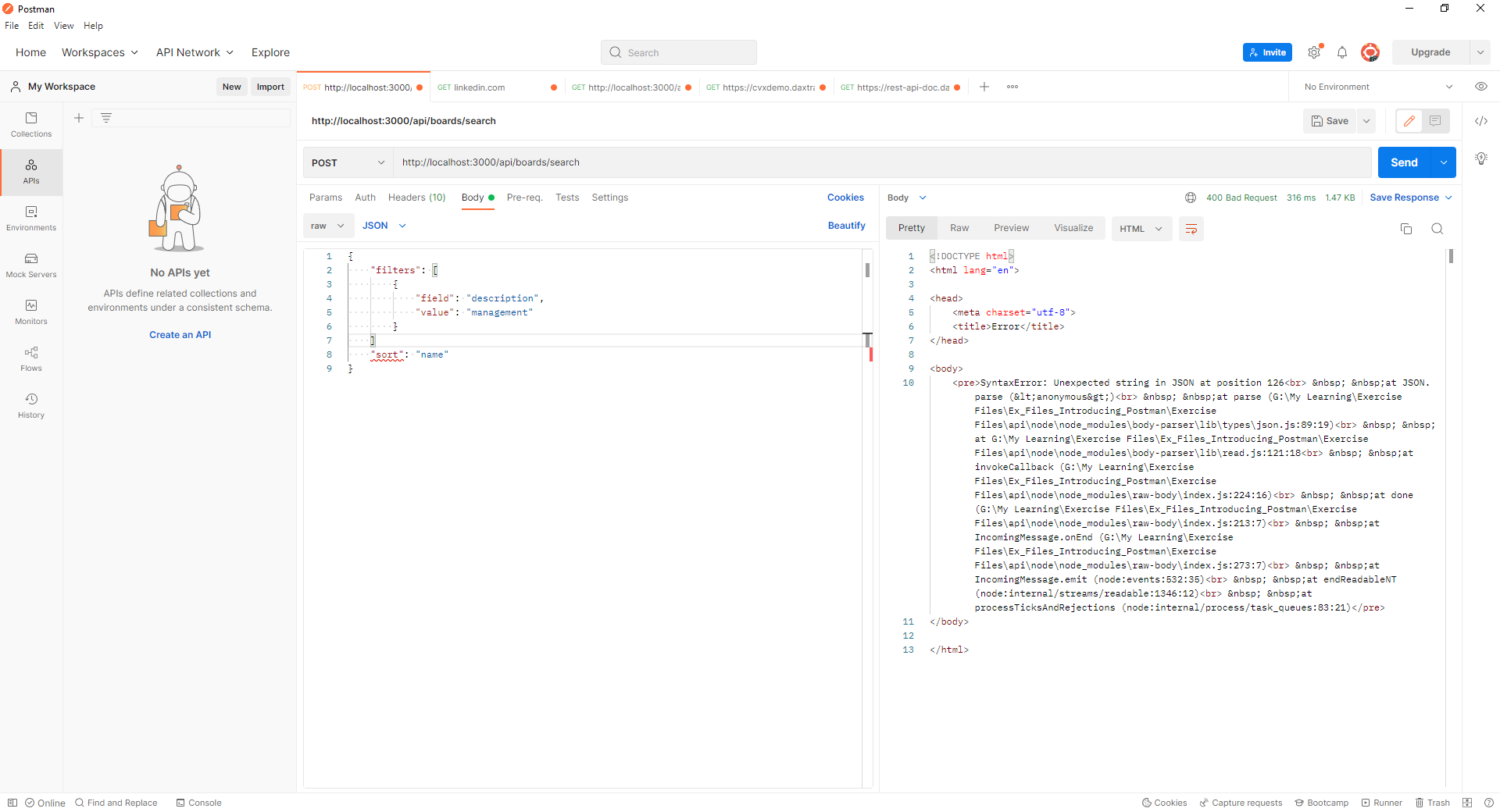
As is often the case with an IDE that tries to locate an error, it has identified the first thing that is incorrect, but it is incorrect because a comma was missing from the previous line. I will insert that and the JSON now looks like that shown in figure 24.
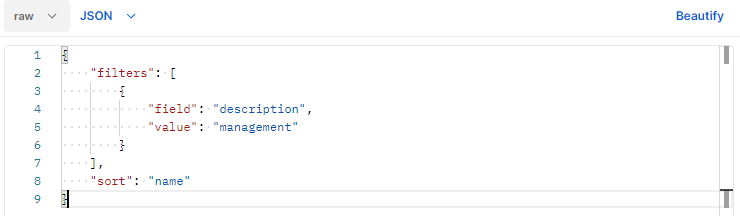
Notice that the word sort is not showing that red squiggly line. If we send the request now, we get the response as shown in figure 25.
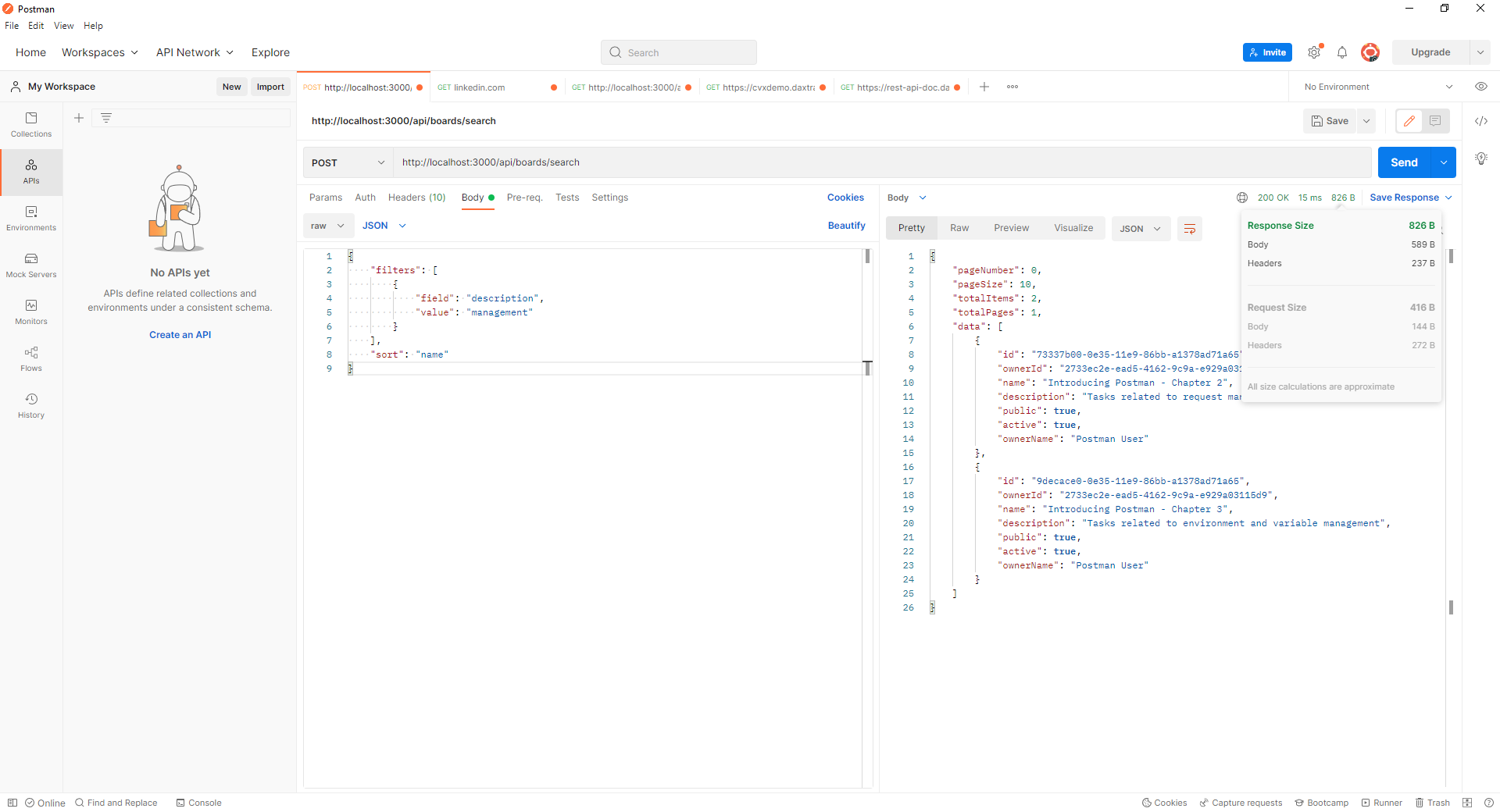
So, we get two results returned. Notice that both of these include the word management in their description and they are sorted alphabetically by name. We can modify the request to, for example, sort by description which will switch the order. If we do that and then modify it again to sort by ownerId, we see something interesting. The results have been resorted into the original order, but the value of ownerId is the same in both. This suggests that there is an underlying order since sorting by ownerId shouldn't have any effect on the order and I would guess that this is simply the order in which the data is sorted within the API.
In any case, it's probably a worthwhile exercise to play around with this request using different sort values, searching for different terms or in different fields.Challenge: Composing a Request
For this challenge, we want to compose a request that will search through a board and return all the results with 2 per page and with a starting point of page 2. The URL for this is
http://localhost:3000/api/boards/{{BOARD_ID}}/tickets
We can insert any available board id into the URL. There are two ways (at least) to get that. From a File Explorer window in Windows, we can navigate to where the API is stored, go into the data folder and open the file, boards.json, using Notepad or some other editor. Alternatively, we can navigate to the folder from the command line and edit the file with the command
notepad boards.json
This will open the file in Notepad and we can get an id such as 632f71a0-0e35-11e9-86bb-a1378ad71a65 and so our URL becomes
http://localhost:3000/api/boards/632f71a0-0e35-11e9-86bb-a1378ad71a65/tickets
It's a good idea to run the request as is before worrying about adding in the query paramaters just to make sure it is working and in this case, it does seem to but it doesn't return any results. We can fix this by opening up the tickets.json which is also in the data folder (inside the API folder) and checking which job boards are actually appearing in the tickets. The id, 4af02b20-0e35-11e9-86bb-a1378ad71a65, appears in 5 tickets so we will use that which means that our URL becomes
http://localhost:3000/api/boards/4af02b20-0e35-11e9-86bb-a1378ad71a65/tickets
As expected, this returns 5 results. We now want to add a couple of query parameters and these are
ps with a value of 2 - this sets the page size to 2.
pn with a value of 2 - this sets 1 as the first page that appears in the results.
Remember that the page numbering starts from 0 so if you use a value of 2 for pn, you will only see 1 result because this is the last page. With 5 results and a page size of 2, the first two pages will have 2 results each and the third will have just 1.
The result of running this request is shown in figure 26. This also shows the query parameters we set.
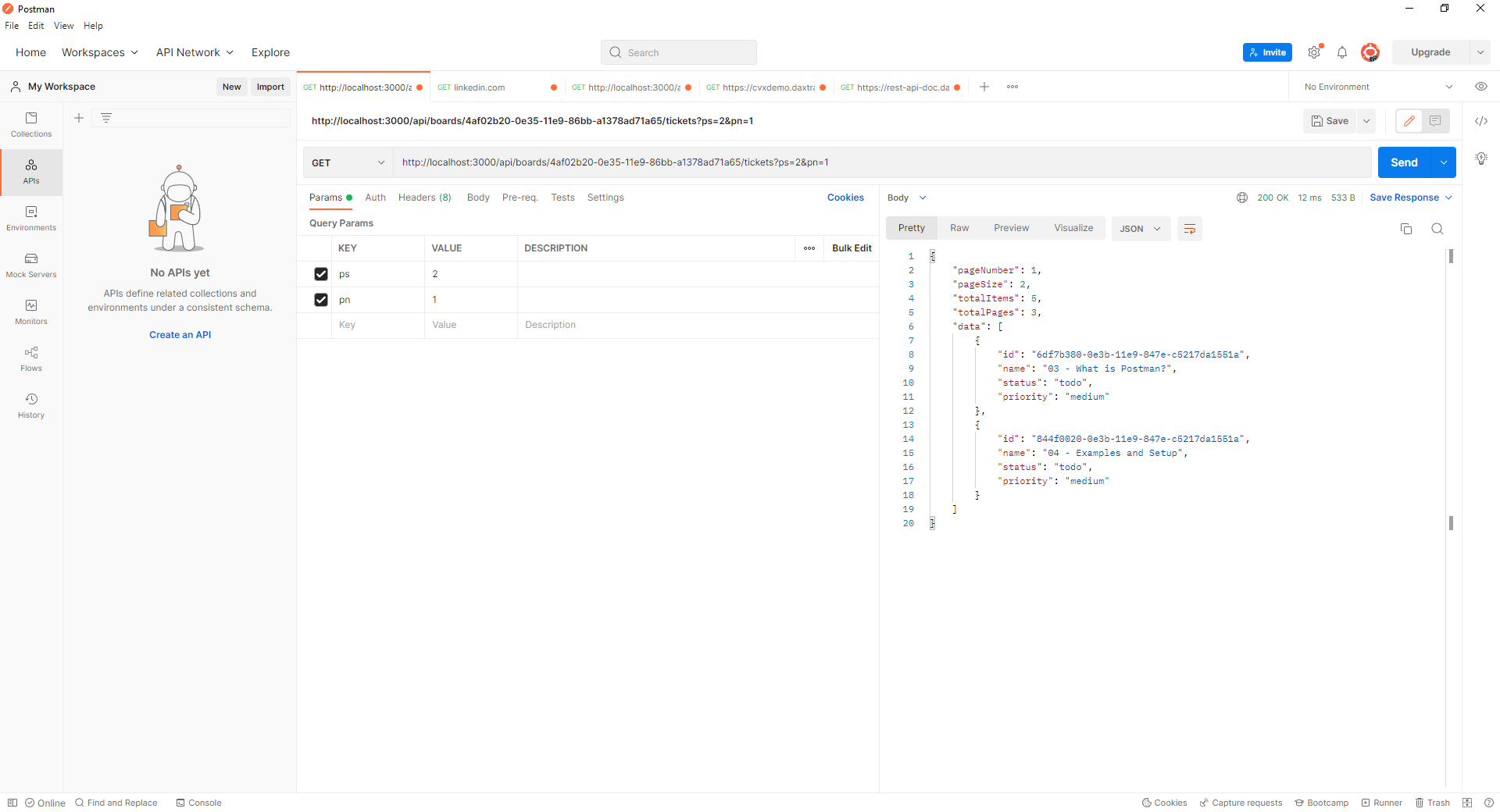